Emmet Cheat Sheet (2017-03) Syntax.1 Child:. Sibling: +. JSX operates in the space between a traditional airline and a private charter company, selling seats on its Embraer 135 and 145 jets but loading passengers out of a private terminal. 📚 The perfect React Cheat Sheet for daily use with a lot of Javascript / JSX snippets! Topics react javascript guideline ressources list reactjs react-redux react-router frontend front-end-development snippets jsx props const router.
What is JSX?
JSX stands for JavaScript XML.
JSX allows us to write HTML in React.
JSX makes it easier to write and add HTML in React.
Coding JSX
JSX allows us to write HTML elements in JavaScript and place them in the DOM without any createElement()
and/or appendChild()
methods.
JSX converts HTML tags into react elements.
You are not required to use JSX, but JSX makes it easier to write React applications.
Let us demonstrate with two examples, the first uses JSX and the second does not:
Example 2
Without JSX:
As you can see in the first example, JSX allows us to write HTML directly within the JavaScript code.
JSX is an extension of the JavaScript language based on ES6, and is translated into regular JavaScript at runtime.
Expressions in JSX
With JSX you can write expressions inside curly braces { }
.
The expression can be a React variable, or property, or any other valid JavaScript expression. JSX will execute the expression and return the result:
Inserting a Large Block of HTML
To write HTML on multiple lines, put the HTML inside parentheses:
Example
Create a list with three list items:

One Top Level Element
The HTML code must be wrapped in ONE top level element.
So if you like to write two headers, you must put them inside a parent element, like a div
element
Example
Wrap two headers inside one DIV element:
React Cheat Sheet 2020
JSX will throw an error if the HTML is not correct, or if the HTML misses a parent element.
Elements Must be Closed
JSX follows XML rules, and therefore HTML elements must be properly closed.
JSX will throw an error if the HTML is not properly closed.
React Cheat Sheet Pdf
Components are the core building block of React apps. Actually, React really is just a library for creating components in its core. (Components & JSX Cheat Sheet)
A typical React app therefore could be depicted as a component tree – having one root component (“App”) and then an potentially infinite amount of nested child components.
Each component needs to return/ render some JSXcode – it defines which HTML code React should render to the real DOM in the end.
Jsx Syntax Cheat Sheet
JSX is NOT HTML but it looks a lot like it. Differences can be seen when looking closely though (for example className in JSX vs class in “normal HTML”). JSX is just syntactic sugar for JavaScript, allowing you to write HTMLish code instead of nested React.createElement(…) calls.
When creating components, you have the choice between two different ways:
- Functional components (also referred to as “presentational”, “dumb” or “stateless” components – more about this later in the course) =>
const cmp = () => { return <div>some JSX</div> }
(using ES6 arrow functions as shown here is recommended but optional) - class-based components (also referred to as “containers”, “smart” or “stateful” components) =>
class Cmp extends Component { render () { return <div>some JSX</div> } }
We’ll of course dive into the difference throughout this course, you can already note that you should use 1) as often as possible though. It’s the best-practice.(Components & JSX Cheat Sheet)
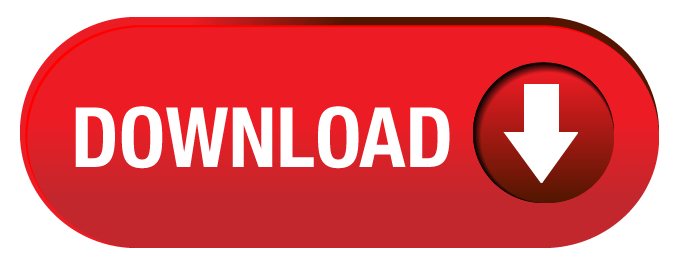